Introduction
With the rise of large language models (LLMs), developers are increasingly looking for ways to customize them for specific tasks, particularly with Retrieval-Augmented Generation (RAG). Ollama provides a seamless way to create, fine-tune, and deploy LLMs while integrating RAG to improve their accuracy and domain specificity.
In this guide, we’ll explore:
- What RAG is and why it matters
- How to build a customized RAG model using Ollama
- How to fine-tune and publish your own LLM model
- Best practices for optimizing performance
What is RAG and Why is it Important?
Understanding Retrieval-Augmented Generation (RAG)
RAG combines the power of pre-trained language models with external knowledge retrieval systems. Instead of relying solely on a model’s static knowledge, RAG fetches relevant documents from an external source (such as a vector database) before generating a response.
This significantly reduces hallucinations and makes responses more factually grounded.
Why Use RAG with Ollama?
- Fine-tune models with external knowledge bases (e.g., PDFs, databases, websites)
- Improve response accuracy by dynamically retrieving relevant information
- Optimize for domain-specific applications such as finance, healthcare, or legal industries
Setting Up Ollama for Custom RAG Implementation
1. Install Ollama
First, install Ollama on your system:
curl -fsSL https://ollama.ai/install.sh | sh
For macOS users with Homebrew, install using:
brew install ollama
2. Download a Base Model
Ollama supports various open-source models (e.g., LLaMA, Mistral, Qwen). Let’s pull a base model:
ollama pull mistral
This downloads the Mistral 7B model, which is a good balance of speed and accuracy.
Building a Customized RAG Model with Ollama
1. Load External Knowledge Sources
To integrate RAG, we need a retrieval system. Here, we use LlamaIndex to index documents:
pip install llama-index
Next, load documents into a vector database:
from llama_index import SimpleDirectoryReader, VectorStoreIndex
# Load documents from a folder
documents = SimpleDirectoryReader("data/").load_data()
# Create an index
index = VectorStoreIndex.from_documents(documents)
# Persist index for future use
index.storage_context.persist()
This will create an indexed vector representation of the knowledge base.
2. Connect Ollama to the RAG Pipeline
To enhance the model’s response, we retrieve relevant documents before generating an answer.
from llama_index import ServiceContext
from llama_index.llms import Ollama
# Load the saved index
index = VectorStoreIndex.from_storage("data/")
# Connect Ollama as the LLM
ollama_model = Ollama(model="mistral")
# Create a service context
service_context = ServiceContext.from_defaults(llm=ollama_model)
# Query the model with RAG
query_engine = index.as_query_engine(service_context=service_context)
response = query_engine.query("What are the latest AI trends?")
print(response)
This pipeline ensures that responses are factually accurate by retrieving relevant documents before generating output.
Fine-Tuning and Publishing Your Custom Model
1. Fine-Tune the Model with Your Data
Ollama allows fine-tuning using custom datasets. First, create a Modelfile
:
touch Modelfile
Edit the file to include:
FROM mistral
PARAMETER temperature 0.7
SYSTEM "You are an AI assistant trained on proprietary data."
# Load custom documents
INSTRUCTION "Use the external database to answer user queries."
Now, build your fine-tuned model:
ollama create my-custom-rag -f Modelfile
This registers your custom RAG model in Ollama.
2. Running Your Customized RAG Model
Start serving the fine-tuned model locally:
ollama run my-custom-rag
To integrate it into a web app, use the Ollama API:
import requests
response = requests.post(
"http://localhost:11434/api/generate",
json={"model": "my-custom-rag", "prompt": "Explain quantum computing"}
)
print(response.json()["response"])
This makes the model available for real-time applications.
Deploying and Publishing Your Model
1. Share Your Model on Ollama Hub
Once satisfied, you can publish the model to make it available to others.
ollama push my-custom-rag
This uploads your model to Ollama’s model hub, making it accessible for anyone to download.
2. Deploying on Cloud Servers
For large-scale deployment, consider hosting the model on a cloud server. Dockerize it for easier deployment:
Create a Dockerfile
:
FROM ubuntu
RUN curl -fsSL https://ollama.ai/install.sh | sh
COPY . /app
WORKDIR /app
CMD ["ollama", "run", "my-custom-rag"]
Build and run the container:
docker build -t ollama-rag .
docker run -p 11434:11434 ollama-rag
Now, your customized RAG model is running in the cloud!
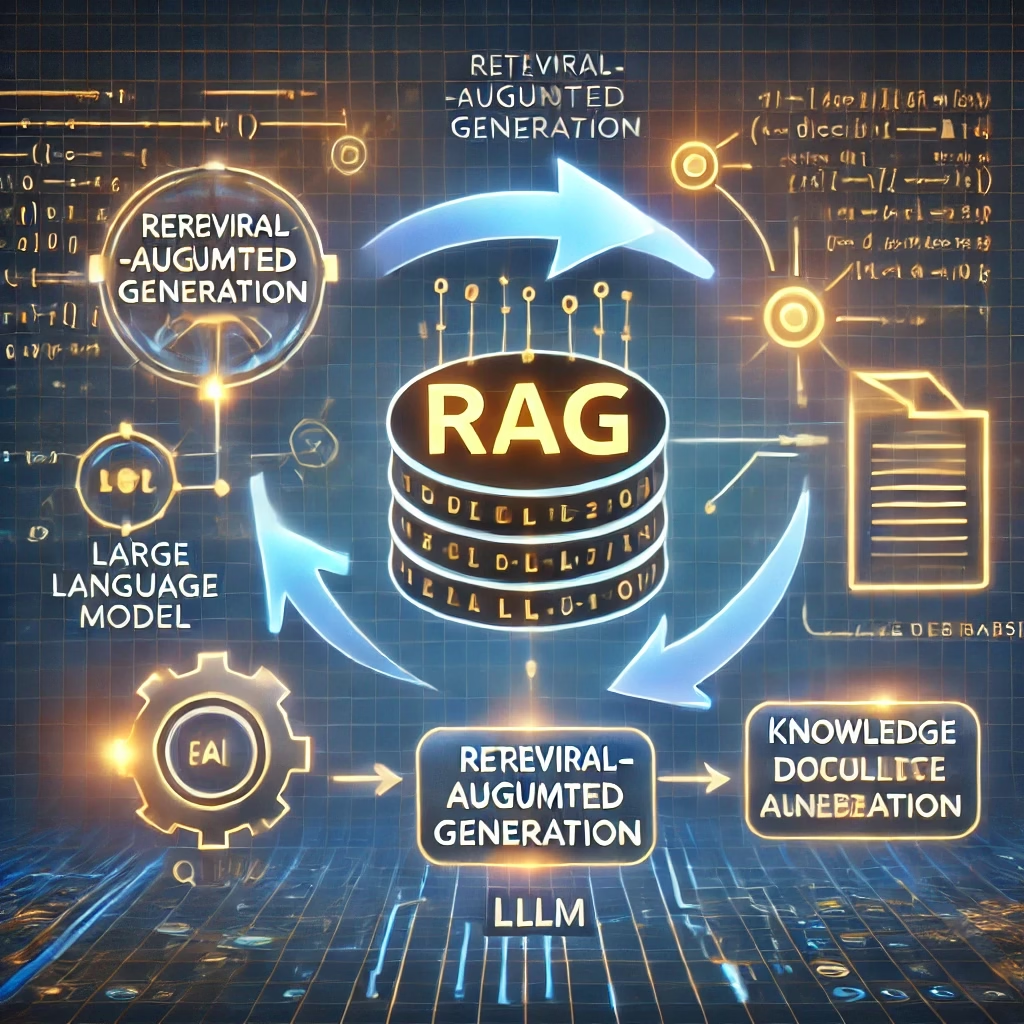
Best Practices for Optimizing Performance
1. Use Efficient Indexing
- Instead of reloading the index every time, save and reuse it
- Use FAISS or ChromaDB for fast vector search
2. Optimize Response Latency
- Reduce the model’s context length
- Adjust temperature and top-k settings in Ollama
3. Regularly Update the Knowledge Base
- Refresh the index periodically with new documents
- Automate updates using cron jobs
Conclusion
By combining Ollama with RAG, you can create highly accurate, domain-specific LLMs. This approach reduces hallucinations and makes AI responses context-aware.
Key Takeaways:
✅ Ollama provides an easy way to customize and publish LLMs
✅ RAG helps retrieve relevant documents before generating responses
✅ Fine-tuning LLMs ensures domain-specific accuracy
✅ Deployment via Docker makes the model scalable
Now, you’re ready to build and publish your own LLM with customized RAG using Ollama! 🚀